Generate a memorizable private key from passwords. Say goodbye to the compromised private key.
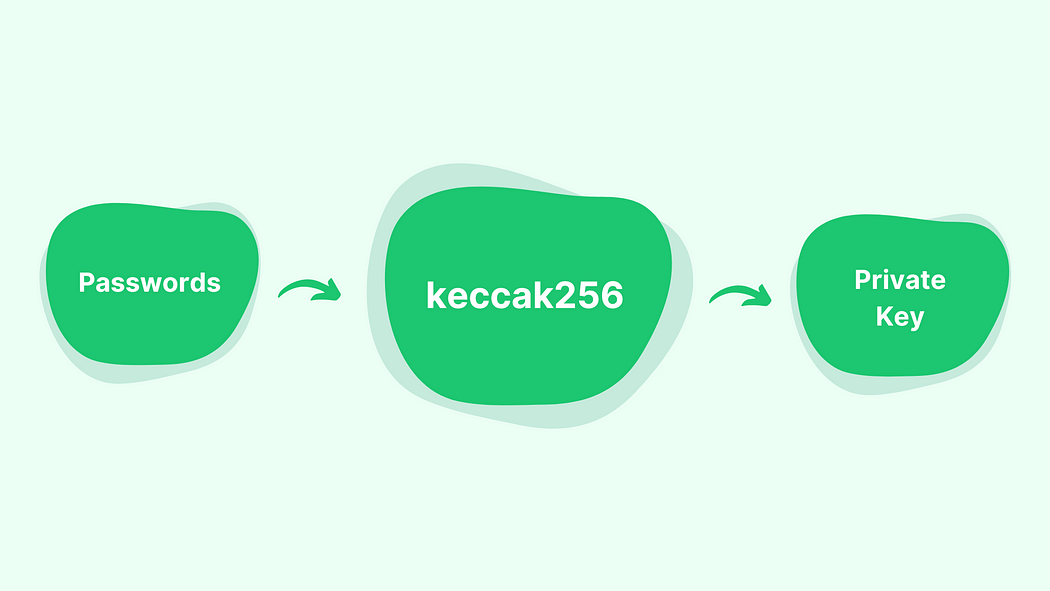
Stop worrying about the compromised or lost private key. You don’t need to worry about hacking or stealing seed phrases or your wife stealing them anymore. Anyway, you must still keep your computer free from being hacked.
Memorizable private key generator website
This tool is available at https://passlet.chom.dev/
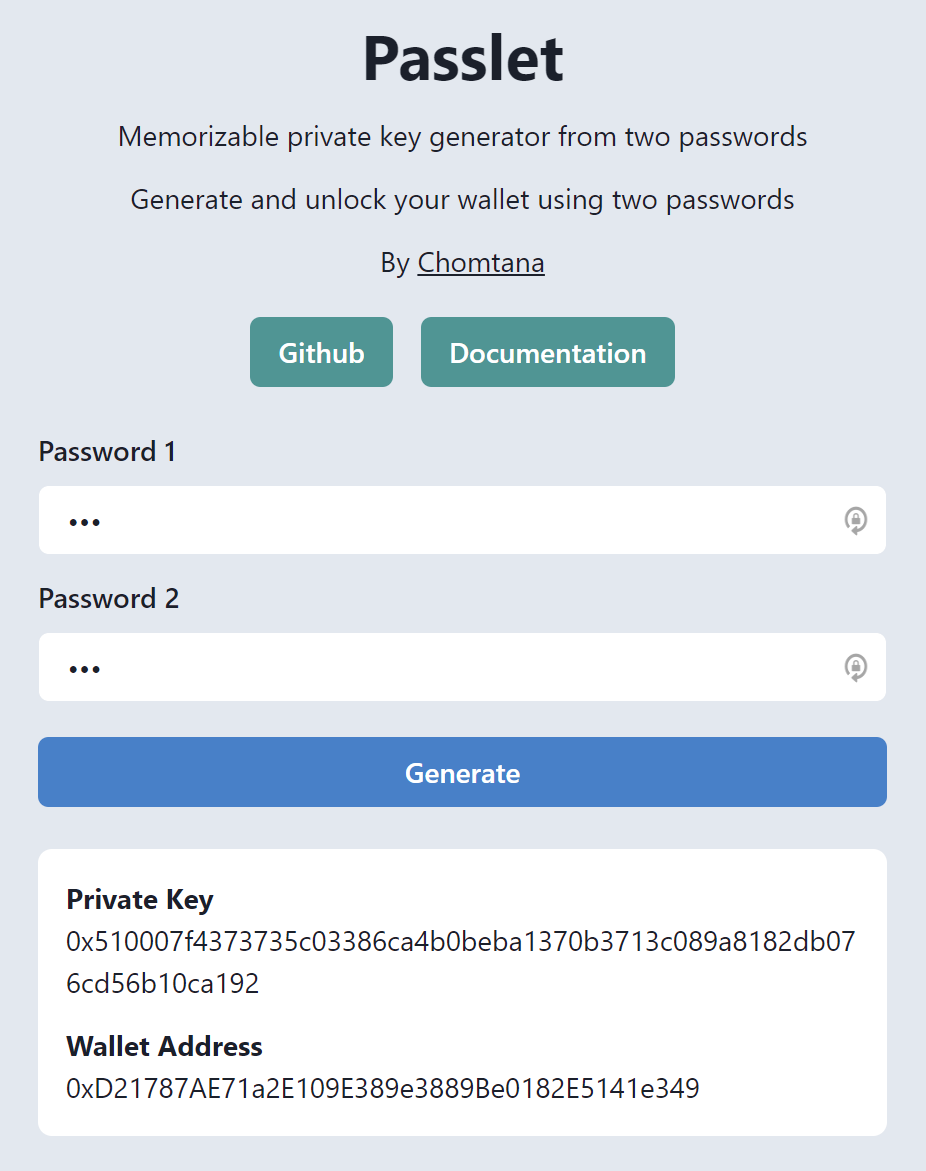
Enter two passwords and press generate to get your private key. You shouldn’t note your passwords anywhere. You must remember your passwords in your brain to maximize security.
Your private key must be kept secret. Keep your private key private from everyone, or you will lose all of your funds. This example shows a private key just for demonstration using an example weak password.
Source code is available at https://github.com/Chomtana/passlet
A private key is just a number.
Many of you know what a private key is but has yet to learn that it is just a random 256-bit number represented in a hexadecimal format. You can generate a private key by randomizing a 256-bit number and converting it to hexadecimal format. You may use eight random 32-bit numbers. Convert each number to hexadecimal format, zero pad them to 8 digits, and concatenate them to form a final 256-bit number. The hexadecimal format of a 256-bit number is a 32-byte number (data type bytes32 in solidity). So, a private key is a random bytes32.
For example, we got eight random numbers: 2663060799, 1097431238, 931084231, 3128907328, 590289273, 1908375297, 28432482, and 2865120899. Next, convert them to hexadecimal format: 9EBB153F, 416978C6, 377F37C7, BA7F5640, 232F1979, 71BF7F01, 1B1D862, and AAC64683. Finally, concatenate them to get a private key: 0x9EBB153F416978C6377F37C7BA7F5640232F197971BF7F0101B1D862AAC64683. Notice that 1B1D862 gets zero-padded to 01B1D862.
You can verify the private key above by importing it to your Metamask. You will have a new account with wallet address 0xd01097b5a5F2AB2bfE42Ed2143060EBa4Be25c0A in your Metamask.
Converting the password to a private key
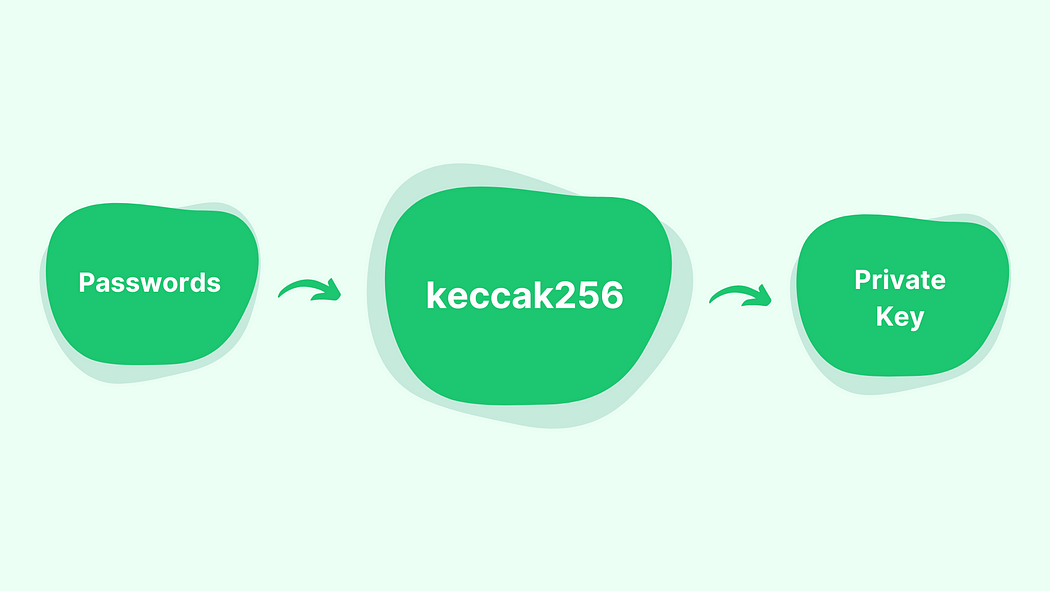
Since the private key is a bytes32, to generate a private key from a password, you must convert the password, a string, to a bytes32. Keccak256 hashing function did this job well. keccak256(password) is a bytes32 that looks randomized. Returned bytes32 number can be used as a private key. Since keccak256 is a hashing function, passing the same password to keccak256 always returns the same private key.
Write a smart contract to generate a memorizable private key from passwords.
// SPDX-License-Identifier: MIT
pragma solidity =0.8.17;
contract PrivateKeyFromPassword {
function getPk(string calldata pw1, string calldata pw2) public pure returns(bytes32) {
return keccak256(abi.encode(pw1, pw2));
}
}
This contract consists of a simple getPk function that takes two passwords and returns a bytes32, a private key. To use this smart contract, you can use the predeployed one on the Binance testnet at 0x2e62409d40132845d4e0cd5E02C6e513Abcc03E5 or deploy it yourself using Remix.
This function will encode two passwords and pass them to keccak256 to generate a bytes32 hash (private key) from these passwords.
To get the wallet address associated with that private key, you should import this private key into your wallet.
Generate a memorizable private key from passwords using javascript or typescript.
const generateKey = useCallback(async () => {
const _privateKey = utils.keccak256(
utils.defaultAbiCoder.encode(["string", "string"], [password1, password2])
);
setPrivateKey(_privateKey);
setWalletAddress(new Wallet(_privateKey).address);
setState(KeyGenerationState.SUCCESS);
}, [password1, password2]);
To generate a memorizable private key from passwords using javascript or typescript, you must pass two passwords entered in the UI to the generateKey function. It will ABI encode two passwords together using utils.defaultAbiCoder.encode([“string”, “string”], [password1, password2]) and then pass the concentrated password into keccak256 to generate the final bytes32 hash used as a private key.
To get the wallet address associated with that private key, you can simply use ethers.js to get the wallet address as usual. Another way is to import this private key to your wallet.
Security concerns in this tool.
Using a password is vulnerable to both rainbow and brute force attacks.
A rainbow attack is an attack that builds a dictionary for the password, public key, and private key of well-known passwords. Hackers will look up the victim’s public key in their rainbow dictionary. If it matches, they will know the victim’s private key immediately. The hacker will immediately drain the fund from the victim’s wallet.
Another way to hack your wallet is to brute force all possible passwords. Although it looks impossible to brute-force a password longer than ten characters, it may be possible in the next ten years. Like the Profanity hack, vulnerability always exists, but it just got hacked in 2022 since hardware capable of performing this hack may only exist since 2022.
Using a single password also makes it vulnerable to password leak problems. If some website leaks your password, your wallet may be hacked immediately. You should use at least two passwords to generate the private key to prevent this. Since most site usually uses only one password.
Most importantly, it would be best if you didn’t use your passwords on any other sites.
Extending this tool to improve security.
To improve security, you should modify the algorithm to create your algorithm dedicated to you. In brief, only you know which algorithm you use to generate your private key.
Since the private key is just a random 256-bit number, you can generate a 256-bit random number from the seed (in this case, passwords) first. Then, you can do anything to that number to get the new random number, such as adding, subtracting, and multiplying. The new random number is a new private key you can use more securely.
An example of modifying this algorithm is to multiply the result with a number. For example, a pair of passwords generate a private key 0x000…1234. We multiply that private key with the number 8765, resulting in a new private key 0x000…1234 * 8765 = 0x000…26F3E64.
Employing your imagination when creating an impenetrable secure algorithm would be best. Your private key will be protected from the previously described rainbow attack and brute force if anyone except yourself cannot guess it.